Unveiling the Power of TypeScript in Vue.js Front-end Development
In the fast-paced world of software development, improving both quality and the developer experience (DX) is key. TypeScript (TS) is an advanced extension of JavaScript that provides powerful tools and features to achieve these goals.
In this article, we'll explore the reasons why integrating TypeScript into Vue.js front-end development can be a game changer.
What is TypeScript?
TypeScript is a superset of JavaScript that introduces static typing and other features to improve code reliability and readability. This piece concentrates on how TypeScript enhances Vue.js development.
Advantages of using TypeScript
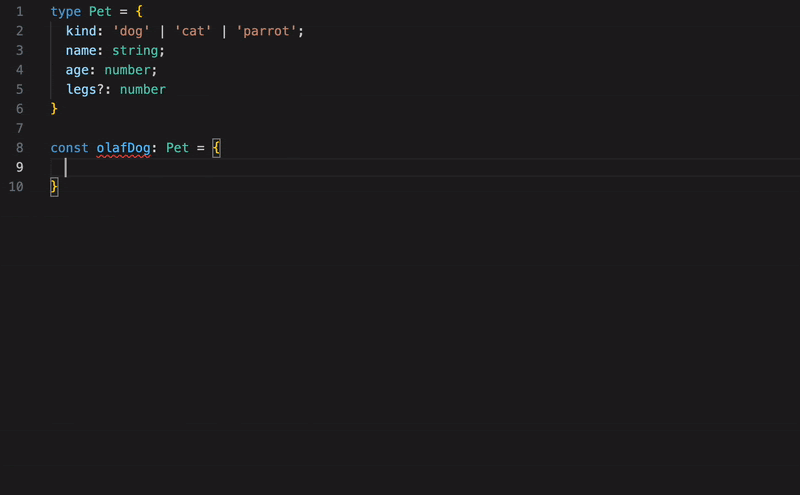
1. Types and safety
With TypeScript, developers can specify types or interfaces for variables and functions, helping to catch type-related errors at coding or compile time. This ensures code safety and reduces debugging time, making the project more stable and reliable.
2. Autocomplete in the IDE
TypeScript greatly improves the developer experience by providing autocomplete functionality in integrated development environments (IDEs). This feature accelerates code writing by suggesting appropriate fields and methods and catching potential errors during the coding process.
3. Easier code changes and refactoring
TypeScript's strong typing allows for easier code changes and refactoring. The IDE can perform mass renaming and find function usage, making it efficient to update the codebase when data type structures change.
4. Improved project understanding
TypeScript enforces defined data types and relationships, making it easier for newcomers to understand the project. This clarity and structure contribute to a smoother onboarding process.
Using TypeScript with Vue.js
Vue.js 3 and its official packages provide exceptional TypeScript support. Developers can leverage features like typed props, emits, and more, enhancing code quality and productivity. Recent updates in Vue 3.3 further improve the Developer Experience, introducing features like imported and complex types support in macros, generic components, and enhanced defineEmits. Personally, I’m also excited about the addition of a new experimental defineModel macro, which allows us to shorten the required code for declaring two-way binding fields. I’m looking forward to seeing the stable release of this feature.
Here is a code example of using some of Vue macros with TypeScript:
<script setup lang="ts" generic="T extends string | number">
// Experimental. RFC#503
const modelValue = defineModel<T>({ required: true });
const props = withDefaults(
defineProps<{
name: string;
min?: number;
max: number;
}>(),
{
min: 1,
}
);
const emit = defineEmits<{
foo: [id: number];
}>();
defineSlots<{
default?: (props: { bar: string }) => any;
}>();
</script>
Tips for using TypeScript in Vue 3
When it comes to optimizing your Vue 3 project with TypeScript, adopting best practices can significantly enhance your development experience. Here are a few indispensable tips that I frequently employ in my work, making the most of TypeScript within the Vue.js ecosystem.
- If you use Visual Studio Code, integrating the Volar (Vue Language Tools) extension is highly recommended. To further enhance IDE performance, consider turning off the built-in TypeScript language service in your project workspace and activating Volar's "Takeover mode." This streamlines the development process by utilizing a unified language service. For more details, refer to Volar Takeover Mode.
- When typing a template ref, ensure the precision of autocomplete and clarity by using types inside brackets of the ref function:
<script setup lang="ts">
import { ref } from 'vue';
const inputRef = ref<HTMLInputElement | null>(null);
</script>
<template>
<input ref="inputRef" type="text">
</template>
- To obtain the type of another component within your Vue 3 project, utilize the InstanceType helper:
type YourComponentInstance = InstanceType<typeof YourComponent>;
- If you need to provide default values for the type-only defineProps declaration, wrap it with withDefaults() compiler macro.
- If you would like to have TypeScript IDE autocomplete for custom environment variables in Vite, you can follow this comprehensive guide: Type-Checking Environment Variables in Vite
- If you use custom meta fields in Vue Router and would like to make them type-safe with autocomplete as well, follow the official guide: Typing Custom Meta Fields in Vue Router
- Streamline testing by implementing a solution for typing reusable wrapper elements in tests using @vue/test-utils. This resolves TypeScript errors and provides valuable autocomplete features for the variable within your IDE:
const mountComponent = () => mount(YourComponent);
let wrapper: ReturnType<typeof mountComponent>;
- TypeScript offers a wealth of utility types such as Omit<>, Pick<>, Record<>, Partial<>, and more. Understanding and utilizing these utility types can significantly enhance your codebase: Discover TypeScript Utility Types.
- TypeScript allows you to use types or interfaces for defining structures. While both are powerful, choosing the appropriate one depends on your specific use case. For insights into the differences and best practices, refer to this comprehensive article: Type vs. Interface in TypeScript. I started my TypeScript journey by combining both types and interfaces. But now I use types all the time. They cover all of my needs in projects and allow me to type not only objects (as interfaces) but everything else as well (e.g. primitive variables, and functions). Furthermore, I use the union types a lot.
- Certain Convention. While TypeScript offers flexibility in naming conventions, it's advantageous to establish a set of rules for naming variables and functions. A simple yet effective convention, such as using the first capital letter, can greatly enhance code readability and maintainability throughout the project.
Personally, I name types with the Pascal case in a singular form. Usually, I split complex types (nested objects, union types) into smaller separate ones for further reusability. All types are stored in individual files so I can import them where I need them (views, components, stores, composables, etc.). It allows to have one dedicated place for all relevant types to a certain entity. Also, placing all type files inside the “types” folder works best for me. - Understanding deeply nested TypeScript errors can be challenging. Consider using the Pretty TypeScript Errors plugin to improve error visibility and make debugging more efficient.
- To improve built-in typings (e.g. JSON.parse(), localStorage, sessionStorage, .filter(Boolean), etc.) I recommend using the library TS Reset.
Potential drawbacks of TypeScript
TypeScript, a powerful tool in Vue.js development, has its challenges. Setting up tsconfig.json can be a bit tricky at first, and it can vary from project to project. However, create-vue can simplify this setup, especially within the Vue ecosystem. Sometimes you need to do 'type gymnastics' to resolve some TS errors that won’t be translated into read JS code. There’s also a compile step into JavaScript code, the taken time which can be precious sometimes; the need to keep both code and types aligned; working with old vanilla JS libraries without type support can be tricky. Despite these challenges, the benefits of using TypeScript in Vue.js development are well worth the effort.
Final Thoughts
In summary, TypeScript significantly improves the developer experience and ensures code reliability in Vue.js development. It's best to start with small experiments and gradually integrate TypeScript into your projects to take full advantage of its capabilities.
I recommend TypeScript for developers with a good understanding of JavaScript. It's very useful for medium to large projects and those with the potential to scale. However, for small applications with minimal logic, sticking with plain JavaScript may be sufficient.
Remember, TypeScript is a tool that will evolve and improve over time. Use it thoughtfully to unlock its full potential and make your development journey both exciting and productive. Happy coding!
LET'S CONNECT
Get a stunning website, integrate with your tools,
measure, optimize and focus on success!
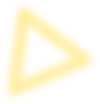
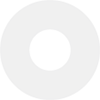

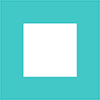
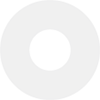
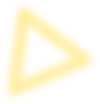